Most places I’ve worked at allow employees to use any of the major browsers to do their work, but mandate an “SOE” that only supports IE, presumably because that generates the most amount of work for us developers. I’d conservatively estimate that 99% of the rendering bugs I’ve had to deal with are only reproducible in IE. (cue one of the thousands of IE joke images… nah, just do a Google Image search, there’s plenty!)
Anyway, we had a number of these rendering issues in Apex on IE8, IE9 and IE10, mainly in edge cases involving some custom CSS or plugins. In some cases I was never able to reproduce the issue until we noticed that the user had inadvertently switched “IE Compatility Mode” on:

We told them to make sure the icon was grey, like this:

– and most of the issues went away.
Since there’s nothing in our Apex application that requires compatibility mode, we would rather the option not be available at all. To this end, we simply add this code to all the Page templates in the application:
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1">
This is added just after the <head>
tag, like this:

Now, the compatibility button doesn’t appear at all – one less choice for users and less bug reports to deal with:

For more information, see this stackoverflow question and read all the answers. Note that it may be better to add this as a header in the response generated by your web server. In our case it was simpler to just add it into the html.
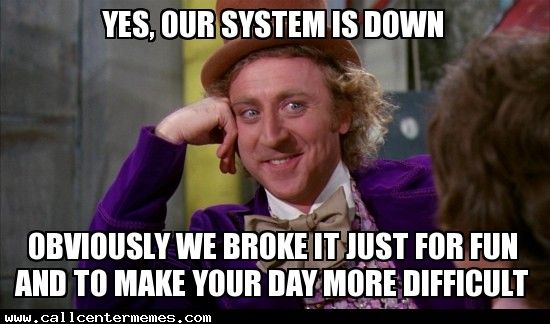
I’ve added this script to our toolbelt for future upgrades. We have a friendly “System is under maintenance, sorry for any convenience” web page that we want to show to users while we run upgrades, and we want it to be shown even if we’re just doing some database schema changes.
So I took the script from here and adapted it slightly, here’s our version:
declare PRAGMA AUTONOMOUS_TRANSACTION;
v_workspace CONSTANT VARCHAR2(100) := 'MYSCHEMA';
v_workspace_id NUMBER;
begin
select workspace_id into v_workspace_id
from apex_workspaces where workspace = v_workspace;
apex_application_install.set_workspace_id (v_workspace_id);
apex_util.set_security_group_id
(p_security_group_id => apex_application_install.get_workspace_id);
wwv_flow_api.set_flow_status
(p_flow_id => 100
,p_flow_status => 'UNAVAILABLE_URL'
,p_flow_status_message => 'http://www.example.com/system_unavailable.html'
);
commit;
end;
/
It uses an autonomous transaction because we want the system to be unavailable immediately for all users while the deployment is running.
Warning: WWV_FLOW_API is an undocumented package so this is not supported.
The opposite script to make the application available again is:
declare PRAGMA AUTONOMOUS_TRANSACTION;
v_workspace CONSTANT VARCHAR2(100) := 'MYSCHEMA';
v_workspace_id NUMBER;
begin
select workspace_id into v_workspace_id
from apex_workspaces where workspace = v_workspace;
apex_application_install.set_workspace_id (v_workspace_id);
apex_util.set_security_group_id
(p_security_group_id => apex_application_install.get_workspace_id);
wwv_flow_api.set_flow_status
(p_flow_id => 100
,p_flow_status => 'AVAILABLE'
);
commit;
end;
/
However, if we run the f100.sql script to deploy a new version of the application, we don’t need to run the “set available” script since the redeployment of the application (which would have been exported in an “available” state already) will effectively make it available straight away.
UPDATE FOR APEX 5.1 and later
The APEX API has been updated with a documented and supported call – APEX_UTIL.set_application_status
to do this which should be used in APEX 5.1 or later.
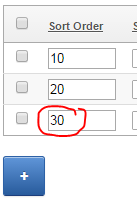
I have a simple tabular form with a numeric “sort order” column. I want the value of this column to be defaulted automatically, based on the maximum value of the rest of the records on the screen. Unfortunately the builtin Apex default type for columns in a tabular form can only be based on an Item, or a PL/SQL expression or function. I didn’t want to make a database call to get the maximum value because the user may have added multiple records which have not yet been saved to the database.
I tried basing the default on a hidden page item which I kept updated based on the values entered, but it seems the tabular form only gets the item’s value on page load and doesn’t re-examine the item’s value when you click the “Add” button. Instead, I had to turn to javascript and jQuery to get the job done.
1. Add a class to the tabular form column by setting the Element CSS Classes column attribute (I’ve used “sortorder” as the class name).
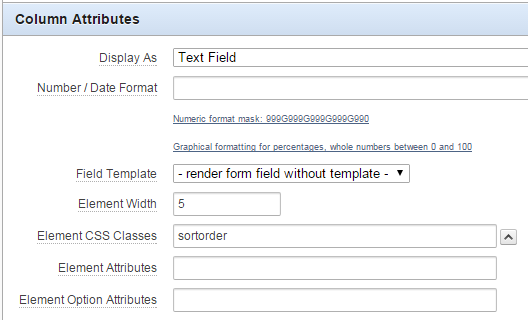
2. Add a javascript function to the page that searches for all the “sortorder” items, calculates the maximum value and adds 10, and assigns it to the last sortorder item on the page.
function setNextSortOrder() {
var highest=0;
$(".sortorder").each(function(){
if (this.value != "") {
highest=Math.max(highest,parseFloat(this.value));
}
});
$(".sortorder").last().val(highest+10);
}
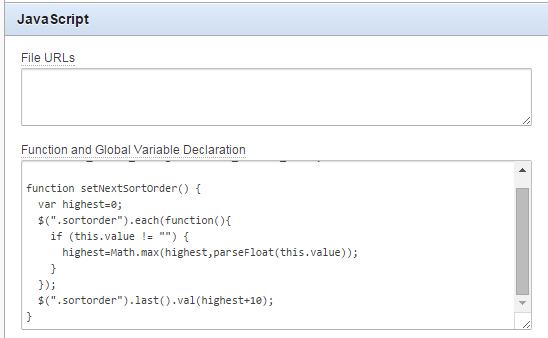
3. Modify the javascript for the “Add” button to call the setNextSortOrder function immediately after adding a row.
javascript:{apex.widget.tabular.addRow();setNextSortOrder();}
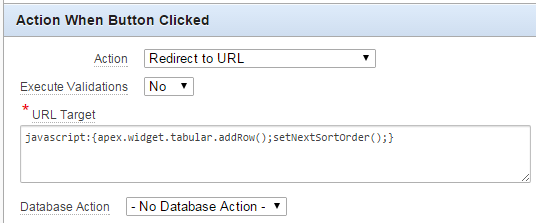
It’s a bit hackish and might not work correctly in some cases but it’ll do for now.
If you are using the APEX built-in Data Loading feature to allow your users to upload CSV files, you may have encountered this error.
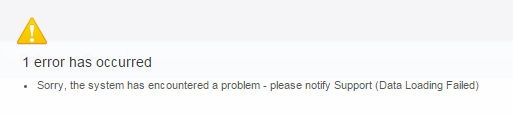
(Note: the error may appear differently in your application as I have built a custom error handling function)
It’s not a particularly useful message, and the logs don’t seem to shed much light on the problem either – reporting only the following:
DATA_LOAD - Final collection is created
...Execute Statement: select 1 from "DEMO"."MY_TABLE" where "RECORD_ID" = :uk_1
Add error onto error stack
...Error data:
......message: Data Loading Failed
......additional_info: ORA-01403: no data found
...
......ora_sqlerrm: ORA-01403: no data found
......error_backtrace:
ORA-06512: at "APEX_040200.WWV_FLOW_DATA_UPLOAD", line 4115
ORA-06512: at "APEX_040200.WWV_FLOW_PROCESS_NATIVE", line 213
ORA-06512: at "APEX_040200.WWV_FLOW_PROCESS_NATIVE", line 262
ORA-06512: at "APEX_040200.WWV_FLOW_PLUGIN", line 1808
ORA-06512: at "APEX_040200.WWV_FLOW_PROCESS", line 453
After trial and error I tracked down one potential cause of this error so I thought I’d share it in case it happens again. I’ll probably come across this again later and forget what the solution was and find this article.
In my case (APEX 4.2.4), the problem was caused by an invalid entry in the Column Name Aliases list of values. I was using a custom List of Values so that alternative names for the columns would be automatically mapped without the user having to select them every time. To do this, I had to edit the List of Values directly to add the alternative names; but I had mistyped one of the Return Values which must map to a real column name on the target table. Whenever I picked this column for an import, I’d get the “Data Loading Failed” error message. Correcting the return value resolved the issue.
In order to stop this happening again, I added the following check to my Apex QA script (this is run whenever the application is deployed):
PROMPT Invalid dataload column mappings (expected: none)
SELECT REPLACE(lt.owner,'#OWNER#',USER) AS owner
,lt.table_name
,le.return_value AS target_column_not_found
,le.list_of_values_name
,le.display_value AS col_alias
,lt.application_id
,lt.application_name
,lt.name AS dataload_definition
FROM apex_appl_load_tables lt
JOIN apex_application_lov_entries le
ON le.lov_id = lt.column_names_lov_id
WHERE NOT EXISTS (
SELECT NULL
FROM all_tab_columns tc
WHERE tc.owner = REPLACE(lt.owner,'#OWNER#',USER)
AND tc.table_name = lt.table_name
AND tc.column_name = le.return_value)
ORDER BY lt.name, le.display_sequence;
If the above query returns any rows, it’ll be a problem.
I’m a morning person, and my mind is usually sharpest on Monday or Tuesday mornings, so these are the best times for me to work on fiddly javascript stuff. Today was one of those mornings and here are the results, just in case I want to refer back to them later on.
I had many items dotted around an Apex application where the user is allowed to enter “Codes” – values that must be uppercase and contain no spaces or other punctuation characters – except underscores (_
) were allowed.
To make things easier for the user, I wanted the page to automatically strip these characters out when they exit the field, instead of just giving validation errors (Note: I still included the validations, but the javascript just makes the process a bit smoother for the user doing the data entry).
My APEX application already has a global .js
file that is loaded with each page, so all I had to do was add the following code to it:
function cleanCode (c) {
return c.replace(/[^A-Za-z0-9_]/g,"");
}
$(document).ready(function() {
//automatically remove non-code characters from
//"edit_code" class
$( document ).on('change', '.edit_code', function(){
var i = "#"+$(this).attr("id");
$(i).val( cleanCode($(i).val()) );
});
});
EDIT: greatly simplified regexp based on the excellent contribution by Jacopo 🙂
EDIT #2: corrected, thanks to Sentinel
Finally, on each “Code” page item, I set the following attribute (or append, if other classes have already been added):
HTML Form Element CSS Classes = edit_code
For code items within a tabular form, I set the following column attribute:
Element CSS Classes = edit_code
If you decide to use an editable tabular form to present a number of records for viewing and/or editing, but you have some users who are only allowed to view the data but not edit it, you’d think you could set the “Readonly” condition on the region; but this condition is only applied to any extra region items you add, not to the editable items within the report itself.
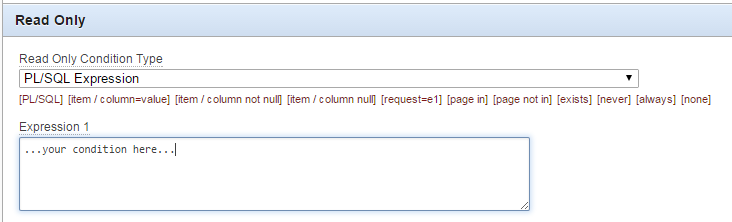
Here’s my tabular form, with the records still editable:
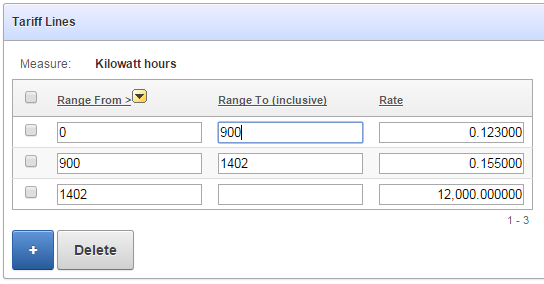
One way to get around this is to have two separate report regions on the page – one is the editable tabular report, the other is an ordinary standard report that doesn’t have any of the edit capabilities – and use conditions to hide one or the other depending on the user’s authorisation.
Another way is to use conditions and jQuery to make all the items in the tabular form readonly:
1. Put a condition on all the buttons (e.g. “Add Row”, “Delete”, “Save”, etc) so they are not shown if the user doesn’t have edit privilege
2. Put the same condition on the Multi-Row processes so that they will not run if the user doesn’t have edit privilege.
3. Set the static ID on the region so jquery can find it:
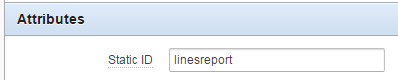
4. Add a Dynamic Action to make all the input items within that region disabled:
Event: Page Load
Authorization Scheme: {Not Editor} (this is just an example where I have an Authorization scheme called “Editor”; alternatively you could set a Condition instead)
True Action: Execute Javascript Code
$("#linesreport input, #linesreport select").prop("disabled",true)
Now, when the page loads, if the user doesn’t have edit privilege the items are rendered readonly, e.g.:
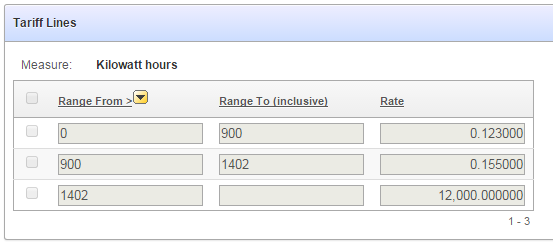
There are other variations on this theme, e.g. we could target the jQuery expression to just the text inputs while still allowing the user to use the checkboxes (e.g. if there was some action that we wanted to allow). Of course, if I wanted to hide the checkboxes completely, I’d just put the authorization on the [row selector] column in the tabular report definition.
When some of my users were using my system to send emails, they’d often copy-and-paste their messages from their favourite word processor, but when my system sent the emails they’d have question marks dotted around, e.g.
“Why doesn’t this work?”
would get changed to
?Why doesn?t? this work??
Simple fix was to detect and replace those fancy-pants quote characters with the equivalent html entities, e.g.:
function enc_chars (m in varchar2) return varchar2 is
begin
return replace(replace(replace(replace(m
,chr(14844060),'“')/*left double quote*/
,chr(14844061),'”')/*right double quote*/
,chr(96) ,'‘')/*left single quote*/
,chr(14844057),'’')/*right single quote*/
;
end enc_chars;
P.S. Stupid wordpress keeps mucking around with my code, trying to replace the html entities with the unencoded versions. In case this doesn’t work, here’s an image of what the above code is supposed to look like:

The business analyst or QA wants to check all the help texts for all items in your apex application – don’t force them to navigate to each page and click on the labels, one by one; instead, give them a spreadsheet to review at their leisure.
Method 1: use the APEX data dictionary viewer
1. Open your application in the APEX application builder
2. Utilities -> Application Express Views
3. Choose APEX Application Page Items
4. Include PAGE_ID, PAGE_NAME, REGION, ITEM_NAME, LABEL, DISPLAY_AS, ITEM_HELP_TEXT
5. Click Filter >
6. Select APPLICATION_ID = <your app id>
7. Select ITEM_HELP_TEXT IS NOT NULL
8. Click Results >
9. Click Download
Method 2: query the data dictionary directly using your tool of choice
select page_id, page_name, region, item_name, label, display_as, item_help_text
from apex_application_page_items
where application_id = :my_app_id and item_help_text is not null
order by page_id, region, display_sequence;
The APEX application I’m working on has a search filter on a report page that looks like this:

The list of values is based on a user-defined “ref codes” table, which includes an option “Show By Default”. This option is currently set on the “Closed” and “Deleted” status and means that transactions with that status will not normally be listed in the report, unless the user explicitly selects either of those statuses, e.g.:

If no checkboxes are selected, the report shows all transactions by default, except for Closed or Deleted transactions.
To indicate this behaviour, I added an asterisk (*) next to the label on those checkboxes. I also wanted some hover text so that a user who has forgotten what the asterisk means can get an idea, e.g.:
<td>
<input type="checkbox" id="P23_FTS_STATUS_7" name="p_v05" value="CLOSED">
<label for="P23_FTS_STATUS_7" title="* not shown by default">Closed*</label>
</td>

However, the default apex Checkbox item doesn’t support putting extra attributes on the generated html labels – so I need to add the hover text by running some javascript after the page is loaded. jQuery to the rescue!
To add the hover text I simply add this to the Execute when Page Loads page attribute:
$("label[for*='P23_FTS_STATUS']:contains('*')")
.attr("title","* not shown by default")
This searches for all label nodes where the “for” attribute contains my item name (“P23_FTS_STATUS”), where the text contains a “*”. It then adds the “title” attribute with my desired value.
I had a registration form in Apex which asks the applicant to enter their Date of Birth in a date item; I then needed to calculate how old they would be at the start of the event, which determines a number of rules, such as whether we need to obtain their parent’s permission.
In my first release I implemented this with a Dynamic Action which ran SQL something like this:
select round(months_between(start_date
,to_date(:P1_DATE_OF_BIRTH,'DD-MON-YYYY'))
/ 12,1)
from events
where event_id = :P1_EVENT_ID;
This worked fine – it takes advantage of Oracle’s builtin support for date arithmetic. However, it was rather slow, because it needs to do a roundtrip to the database to run the query and return the result.
I wanted a pure javascript implementation to avoid the roundtrip, but my initial searches came up with a number of sub-par solutions involving extracting the year and month portions and applying simple arithmetic which did not take into account leap years.
Instead, I’ve gone with an easier solution taking advantage of the moments javascript package.
- Add the path to moment.min.js in the File URLs attribute of the page. You could get your own local copy or point to the relevant file from a cdn: http://cdnjs.com/libraries/moment.js/
- Add a function to the Function and Global Variable Declaration attribute of the page, which uses the moments object to convert the strings into date objects, and then call the diff method to get the number of years as a floating-point number, e.g.:
function getAge() {
var e = moment($v("P1_EVENT_DATE"),"YYYYMMDD")
,dob = moment($v("P1_DATE_OF_BIRTH"),"DD-MMM-YYYY");
return e.diff(dob,'years',true).toFixed(1);
}
- Add a Dynamic Action to the Date of Birth item which calls getAge() and sets the value of the Age display item.
The result is a much quicker response and less load on the database. This is an intentionally simple example, you could do it in different ways to suit your situation (e.g. if you have multiple date items you need to handle on the same page, you might pass them as parameters to the function).
The moments javascript package has an impressive list of features, including pretty-formatting a duration (e.g. a client-side version of the SINCE format e.g. “3 years ago”) documented here.