Conditionally Required Floating Item
An item in the Universal Theme using the Optional – Floating template looks like this:

An item using the Required – Floating template looks like this:

In addition, if the item is required we would most probably set the Value Required attribute to Yes. What if the item is sometimes required but not always? How do we create a Conditionally Required field?
Firstly, we would make sure there is a Validation on the field that checks that the value is provided if required. This way, regardless of what the form may or may not send to the database, it is validated appropriately.
Secondly, to indicate to the user that the item is required or optional, based on the value of another item, we can use a Dynamic Action that sets the required
item property (this triggers the client-side validation) and adds or removes the is-required
class from the item’s container (this shows the little red “required” indicator on the page).
For example, let’s say that whether item P1_COST_CENTRE
is required or not is dependent on whether a hidden item, P1_COST_CENTRE_REQUIRED
, has the value 'Y'
.
- Create a Dynamic Action
- Event: Change
- Selection Type: Item(s)
- Item(s):
P1_COST_CENTRE_REQUIRED
- Client-side Condition Type: Item = Value
- Item:
P1_COST_CENTRE_REQUIRED
- Value:
Y
- Create a True Action: Execute JavaScript Code
var item = $("#P1_COST_CENTRE"); item.prop("required",true); item.closest(".t-Form-fieldContainer").addClass("is-required");
- Create a False Action: Execute JavaScript Code
var item = $("#P1_COST_CENTRE"); item.prop("required",false); item.closest(".t-Form-fieldContainer").removeClass("is-required");
The above code works for all item templates (“Optional”, “Optional – Above”, “Optional – Floating”, etc.) in the Universal Theme; I’ve tested this on APEX 18.2 and 19.1.
Note: this is custom code for the Universal Theme, so it may or may not work for other themes; and might stop working in a future revision of the theme.
Plugins
UPDATE 29/7/2019: I’ve created some simple Dynamic Action plugins (for APEX 18.2 and later) to implement this, if you’re interested you can download them from here:
To use these plugins, select them as an Action to take on a Dynamic Action:
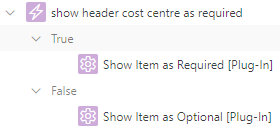
EDIT 29/7/2019: modified to use a better method to find the container div.
Older Themes
In other themes, the way that a required field is rendered is different. For example, in Theme 26 (Productivity Applications) the label for a required item is rendered in bold, along with a red asterisk; if the item is optional, no red asterisk is rendered. The way to make an item conditionally mandatory in this theme is:
- Set the item to use the Required template (so that the red asterisk is rendered).
- In the Dynamic Action JavaScript, execute the following if the item should be optional:
var itemLabel = $("label[for='P1_COST_CENTRE']"); itemLabel.removeClass("uRequired"); itemLabel.addClass("uOptional");
To make the item required again:
var itemLabel = $("label[for='P1_COST_CENTRE']"); itemLabel.removeClass("uOptional"); itemLabel.addClass("uRequired");
Stuart
16 October 2019 - 8:24 am
Hello Jeffrey,
I can get this to work fine on a normal text box but I need it to work on a text field with auto complete.
The required label works fine but the physical ‘required’ does not.
PS. I am just using the js – not the plugin.
Thank you
Scott VK
7 March 2020 - 8:02 am
You Rock, Jeff! Thank You. Conditional Red Dog-Ear is exactly what I was looking for.
Taha BK
16 July 2020 - 10:16 pm
Man, thanks a lot for this. It works like a charm. I had to add a validation to make my radio group truly required, but it works nicely. Thanks again!
Frank
22 October 2020 - 1:59 am
Thanks, very good 😉
It works on select list, but not on radio groups.
Any idea why.
best regards
Frank
Jeffrey Kemp
24 October 2020 - 7:44 pm
Hi Frank,
It works in the latest version of APEX 20.2 – https://apex.oracle.com/pls/apex/jk64/r/conditionally-required-items/home
Which version are you using? Maybe there’s an issue with the template.
Marc
17 November 2020 - 6:20 am
How do I do this for multiple fields at once? Or do I have to do separate dynamic actions for each field?
Jeffrey Kemp
17 November 2020 - 7:41 am
Hi Marc,
If you want one dynamic action to affect multiple elements, set a Class on each item (Advanced -> CSS Classes), e.g. “condRequired”, and on the Action set Selection Type to jQuery Selector, with an expression like “.condRequired”.
Of course, if you want a different rule for different elements, you will still need separate dynamic actions in each case; in more complex cases I would revert to writing some custom JavaScript instead.
Jeff
Marc
17 November 2020 - 11:12 pm
Thanks for the reply. So, after doing this, I still need to use JavaScript code for the True/False actions right? What would that code look like? I tried using just the last two lines of your code (and under ‘Affected Elements’, I chose the jQuery Selector), but that didn’t work:
item.prop(“required”,true);
item.closest(“.t-Form-fieldContainer”).addClass(“is-required”);
Thanks.
Jeffrey Kemp
18 November 2020 - 5:12 pm
Hi Marc, no, if you are using the plugin you don’t need any javascript. If you choose jQuery Selector, you need to enter a jQuery selector expression, e.g.
.my-item-class
Madelein van Duuren
18 November 2020 - 2:03 pm
This is an excellent piece of code. Helped me immensely where I optionally set username and password fields as required when users have accepted terms and conditions to register an account online.
Everton Costa
24 April 2021 - 12:36 am
Thanks a lot this helped me a lot.
In my case I had a field called “DEACTIVATION_DATE , when this field was not null another field called “DEACTIVATION_REASON” becomes Enable and required.
I had a Dynamic action on “DEACTIVATION_DATE” like this
Event: Change
Selection Type: Item(s)
Item(s): P9002_DEACTIVATIONDATE
Client-side Condition Type: Item is not null
So I have created some actions
A true Action wich enables P9002_DEACTIVATION_REASON
Another true Action that I just added and adapted your script and works perfectly.
A false Action wich disables P9002_DEACTIVATION_REASON
Another false action with your script adapted.
Now I want to change Item template (not attribute) for optional but I didn´t know how to do without creating another Item with optional template and changing it dynamicaly on P9002_DEACTIVATION_DATE change event.
Do you have any tip ? Forgive me I am just 20 years experienced Oracle Forms guy migrating to Apex I have almost none knowledge on Js and css .
Jeffrey Kemp
24 April 2021 - 12:55 am
Hi Everton,
Sorry but it’s not clear what you’re trying to achieve or what is not working. I can probably help – and the code required will possibly be different depending on what version of APEX you’re using and what theme.
Jeff
Everton Costa
24 April 2021 - 4:46 am
Reading again what I wrote looks a bit confused indeed.
In resume, your code worked to me, I am enabling a field dynamicaly depending on another field value.
Next step was switch classes display_only and text_field in according with those changes I did before.
After send previous reply I done by myself, another time adapting your solution, thank you again.
saverio
6 July 2021 - 12:22 am
Hi Jeffrey,
I can’t apply your suggestion in an old application running on Apex 4.2.4 with the 13. Classic Blue theme.
I’m using the following javascript code in DA to get the indicator (*) for required label :
var itemLabel = $(“label[for=’P31_OS_CLASS’]”);
itemLabel.removeClass(“t13OptionalLabelwithHelp”);
itemLabel.addClass(“t13OptionalLabelwithHelp”);
But unfortunately it doesn’t work.; do you have any suggestions?
thank you
regards
saverio
Jeffrey Kemp
6 July 2021 - 8:09 am
Hi saverio,
I don’t have access to a 4.2.4 system so I can’t check. I don’t understand why you are removing and adding the same class though? You’ll need to inspect the dom and see what exactly the class names are on required vs optional items.
Jeff
Suresh
17 January 2022 - 12:36 pm
Thanks Jeffery, I tried to use above no luck but when use the below it works
//$(‘#Pxx_PAGE_ITEM_CONTAINER’).addClass(“is-required”);
//$(‘#Pxx_PAGE_ITEM’).prop(“required”,true);
Stephan
13 September 2022 - 5:41 pm
Hi Jeffrey,
we’re running on 21.2.5.
But obviously there is a differing behaviour with Popup LOV-Items.
Now something weird – that’s what i did:
1. Declaring LOV and setting “Value required” to TRUE. Template “Required – floating”. Submitting the page brings up “must have some value” error message. Well done!
2. Declaring second LOV and setting “Value required” to FALSE.
Using a JS-Function doing exactly the same as you did above will change all Form-Items, Date-Items, Popup LOV and Select-Lists and display them as mandatory items.
Now when page submits, all the item validation works for all items except the Popup LOV type.
Now errors for missing values are displayed except for those items, that are of type Popup LOV. This is an obligatory behaviour of mandatory items and does not work.
Any idea on this?
Regards
Stephan
Jeffrey Kemp
13 September 2022 - 6:50 pm
Hi Stephan,
You’ll still need to implement server-side validation as always – if the item isn’t defined as Value Required, you’ll need to add a Validation for it.
Steffen Clauß
28 March 2023 - 4:46 pm
Hi Jeffrey.
For APEX 21.2 (and above) I did the following:
let item = $("#MY_ITEM");
item.prop("required",true);
item.closest(".t-Form-fieldContainer").addClass("is-required");
item.find(".t-Form-inputContainer").prepend('');
Regards
Steffen
Jeffrey Kemp
28 March 2023 - 4:56 pm
Thanks Steffen!
Poorva
17 January 2024 - 6:50 pm
Hi Jeffrey,
Am using Apex 23.2.1. I need to make the items which is optional-floating by default, and on page load based on some setup parameters I need to change the items to Required-floating. The above code makes the item as required. But it is not displayed in UI as required item. When I submit the page it is firing the required validation. But required indicator is not coming in UI.
Note: I tried the above code, for the item which is required-floating by default and making it required/not-required based on some condition which displays the * sign if its set required to true and not displaying the * sign if required is set to false.
Any Idea on this?
Thanks & Regards,
Poorva
Poorva
17 January 2024 - 8:30 pm
Hi Jeffrey,
I am using Apex 23.2.1. I tried using the above JS code to make the item required on page load(based on some setup parameters) which is optional-floating by default.
It is changing the item as required(on submit it is throwing validations) but in UI it is not showing the required indicator.
I am new to apex. Am i missing anything?.
Thanks & Regards,
Poorva